正则表达式-RegExp
特殊符号含义
^ 表示输入开始
$ 表示输入的结束
* 表示任意个字符(包括0个)
+ 表示至少一个字符
? 表示0个或1个字符
[] 表示范围
() 表示想要提取的分组
. 表示任意一个字符
\b 匹配单词边界
\B 匹配非单词边界
(?:x) 非捕获括号,匹配整个x
(?:foo)+ 匹配一个或多个foo
另,
量词例如*、+、?或{}的后面加上?,表示切换至非贪婪模式(匹配尽可能少的字符)。
RegEx对象方法
test() 用于测试给定字符串是否符合条件
exec() 如果正则表达式中定义了组(含圆括号),可以在RegEx对象上用exec()方法提取出子串。
正则表达式中需要转义的特殊字符:
^ $ | ? . * + { } [ ] ( ) / \
示例:提取网站名
var re = /www\.(.+?)\.com/g;//非贪婪模式
var str = "www.baidu.com www.google.com www.baidu.com";
console.log(re.exec(str));//["www.baidu.com", "baidu"]
console.log(re.exec(str));//["www.google.com", "google"]
console.log(re.exec(str));//["www.baidu.com", "baidu"]
String的方法
match()、search()、replace()、split()
String方法进行正则匹配会忽略lastIndex。
exec()和match()方法返回的都是数组,但exec()返回值还包括index和input两个属性。
var pattern = /(www)\.(baidu)\.(com)/g;
var str2 = 'www.baidu.com www.google.com www.baidu.com';
console.log(pattern.exec(str2));
console.log(pattern.lastIndex); //13
//console.log(str2.match(pattern));
console.log(pattern.exec(str2));
console.log(pattern.lastIndex);
console.log(pattern.exec(str2));
console.log(pattern.lastIndex);
console.log(str2.match(pattern));
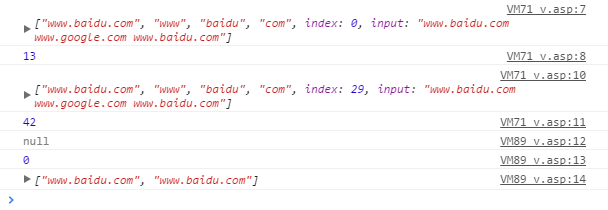
正则表达式标志
g 全局搜索
i 不区分大小搜索
m 多行搜索
y 执行粘性搜索
g 全局搜索
查找所有匹配而非查找到一个后立即返回,查找到第一个匹配字符串后从下一个位置lastIndex开始继续查找。
在全局搜索下,每次匹配成功都会更新lastIndex的值。
var re = /o/g; //全局匹配
console.log(re.lastIndex); //0,lastIndex属性的初始值为0
console.log(re.test("foo")); //true,匹配了第二个字符
console.log(re.lastIndex); //2,lastIndex属性的值被设置为2,也就是第二个字符之后
console.log(re.test("foo")); //true,从第二个字符(lastIndex属性的值)之后开始匹配,匹配了第三个字符
console.log(re.lastIndex); //3,lastIndex属性的值被设置为2,也就是字符串的末尾
console.log(re.test("foo")); //false,已经没有字符了,匹配失败.
console.log(re.lastIndex); //0,lastIndex属性的值被重置为0
console.log(re.test("foo")); //true,又一次重新开始匹配
console.log(re.lastIndex); //2,一直循环下去
re.lastIndex = 3; //手动修改为3
console.log(re.test("foo")); //false,从第三个字符后开始匹配,所以匹配失败
y 执行粘性搜索 ES6特性
“y” 标志指示,仅从正则表达式的 lastIndex 属性表示的索引处为目标字符串匹配(并且不会尝试从后续索引匹配)。
在进行/y匹配时,lastIndex不会自动归零,在正则表达式中会隐式地加入开头元字符^,使得正则从锚定lastIndex位置开始匹配。详情参见
JavaScript:正则表达式的/y标志 和RegExp.prototype.sticky
var regex = /^foo/y;
regex.lastIndex = 2;
regex.test("..foo"); // false - 索引2不是字符串的开始
var regex2 = /^foo/my;
regex2.lastIndex = 2;
regex2.test("..foo"); // false - 索引2不是字符串或行的开始
regex2.lastIndex = 2;
regex2.test(".\nfoo"); // true - 索引2是行的开始
参考/引用: